isaeken/jquery-waves
A simple & lightweight waves effect plugin
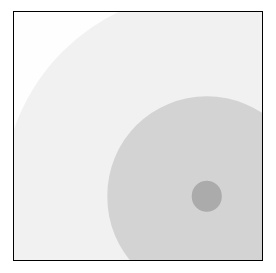
Installation
You can install jquery-waves plugin using npm or include directly files
Install with using npm
npm install animejs
npm install jquery-waves
or include files
<link rel="stylesheet" href="/your/servers/assets/path/css/jquery-waves.min.css">
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/animejs/3.2.1/anime.min.js"></script>
<script src="/your/servers/assets/path/js/jquery-waves.min.js"></script>
Documentation
Creating Waves Effect
You can create waves using classes for example:
or you can create wave effect with attributes for example:
<button class="waves-effect">Button</button>
<button data-waves>Button</button>
Wave effect with background color
<button data-waves data-waves-background-color="rgba(255, 0, 0, 0.35)">Button</button>
Colored Wave With Classes
<button class="waves-effect">Default</button>
<button class="waves-effect waves-light bg-black">Light</button>
<button class="waves-effect waves-red">Red</button>
<button class="waves-effect waves-yellow">Yellow</button>
<button class="waves-effect waves-orange">Orange</button>
<button class="waves-effect waves-purple">Purple</button>
<button class="waves-effect waves-green">Green</button>
<button class="waves-effect waves-teal">Teal</button>
Rainbow Wave
<button data-waves data-waves-background-color="RANDOM">Button</button>
Wave effect like mdbootstrap ripples
<button class="waves-effect">Default</button>
<button class="waves-effect waves-light bg-black">Light</button>
<button class="waves-effect waves-red">Red</button>
<button class="waves-effect waves-yellow">Yellow</button>
<button class="waves-effect waves-orange">Orange</button>
<button class="waves-effect waves-purple">Purple</button>
<button class="waves-effect waves-green">Green</button>
<button class="waves-effect waves-teal">Teal</button>
Wave effect with opacity
<button data-waves data-waves-background-color="red" data-waves-opacity="0.35">Button</button>
Wave effect with duration
Default
<button data-waves data-waves-duration="600" data-waves-hide-duration="800">Button</button>
Fast
<button data-waves data-waves-duration="300" data-waves-hide-duration="400">Button</button>
Slow
<button data-waves data-waves-duration="800" data-waves-hide-duration="1200">Button</button>
Ripples in box
.box {
position: relative;
display: inline-block;
margin: 0.1rem;
cursor: pointer;
width: 250px;
height: 250px;
border: 1px solid black;
}
<div class="box waves-effect">This is a box</div>
<div class="box bg-black waves-effect waves-light">This is a box</div>
<div class="box waves-effect waves-red">This is a box</div>
<div class="box waves-effect waves-yellow">This is a box</div>
<div class="box waves-effect waves-orange">This is a box</div>
<div class="box waves-effect waves-purple">This is a box</div>
<div class="box waves-effect waves-green">This is a box</div>
<div class="box waves-effect waves-teal">This is a box</div>
This is a box
This is a box
This is a box
This is a box
This is a box
This is a box
This is a box
This is a box
<div class="box waves-effect waves-material">This is a box</div>
<div class="box bg-black waves-effect waves-material waves-light">This is a box</div>
<div class="box waves-effect waves-material waves-red">This is a box</div>
<div class="box waves-effect waves-material waves-yellow">This is a box</div>
<div class="box waves-effect waves-material waves-orange">This is a box</div>
<div class="box waves-effect waves-material waves-purple">This is a box</div>
<div class="box waves-effect waves-material waves-green">This is a box</div>
<div class="box waves-effect waves-material waves-teal">This is a box</div>
This is a box
This is a box
This is a box
This is a box
This is a box
This is a box
This is a box
This is a box
Create wave effect with programmatically
<div id="rippleContainer" data-waves-background-color="random" class="box" style="display: block"></div>
<button id="addRipple">Click me to add ripple</button>
<script>
$('#addRipple').click(function () {
$('#rippleContainer').rippleAnimation();
});
</script>
<div id="elementRipple" class="box" style="display: block">Click me</div>
<button id="removeRipple">Click me to remove ripples</button>
<script>
var ripples = [];
$('#elementRipple').click(function () {
var ripple = $(this).ripple(
50, // x position
80, // y position
);
ripples.push(ripple);
});
$('#removeRipple').click(function () {
$.each(ripples, function (index, ripple) {
$('#removeRipple').hideRipple(ripple);
});
});
</script>
Click me
<div id="elementRippleCenter" class="box" style="display: block">Click me</div>
<button id="removeRippleCenter">Click me to remove ripples</button>
<script>
var ripples = [];
$('#elementRippleCenter').click(function () {
var ripple = $(this).ripple();
ripples.push(ripple);
});
$('#removeRippleCenter').click(function () {
$.each(ripples, function (index, ripple) {
$('#removeRippleCenter').hideRipple(ripple);
});
});
</script>
Click me